TypeScript Support
ReactPixi gives you full type support.
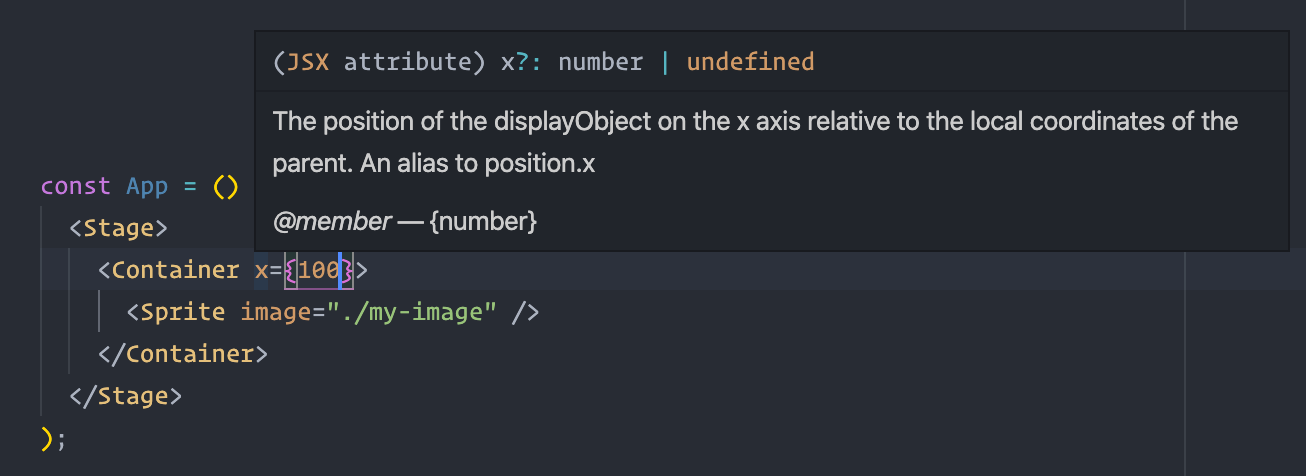
PointLike types
The props position
, scale
, pivot
, anchor
and skew
are PointLike types.
type PointLike =
| { x: number; y: number }
| Point
| ObservablePoint
| number
| [number]
| [number, number];
Example:
import { Sprite } from '@pixi/react';
import { Point } from 'pixi.js';
<Sprite anchor={{ x: 0.5, y: 0.5 }} />
<Sprite anchor={new Point(0.5, 0.5)} />
<Sprite anchor={0.5} />
<Sprite anchor={[0.5]} />
<Sprite anchor={[0.5, 0.5]} />
Source types
Apply source directly to a component. Source types are available for:
<Sprite>
<Text>
<NineSlicePlane>
<TilingSprite>
<SimpleRope>
<SimpleMesh>
<AnimatedSprite>
type Source = {
image?: ImageSource;
video?: VideoSource;
source?:
| number
| ImageSource
| VideoSource
| HTMLCanvasElement
| Texture;
};
Example:
<Sprite image="./my-image.png" />
<Sprite video={document.querySelector('#video')} />
Custom Components
Example:
import { PixiComponent } from '@pixi/react';
import { Graphics } from 'pixi.js';
interface RectangleProps {
x: number;
y: number;
width: number;
height: number;
color: number;
}
const Rectangle = PixiComponent<RectangleProps, Graphics>('Rectangle', {
create: () => new Graphics(),
applyProps: (ins, _, props) => {
ins.x = props.x;
ins.beginFill(props.color);
ins.drawRect(props.x, props.y, props.width, props.height);
ins.endFill();
},
});
const App = () => (
<Stage>
<Rectangle x={100} y={100} width={100} height={100} color={0xff0000} />
</Stage>
);
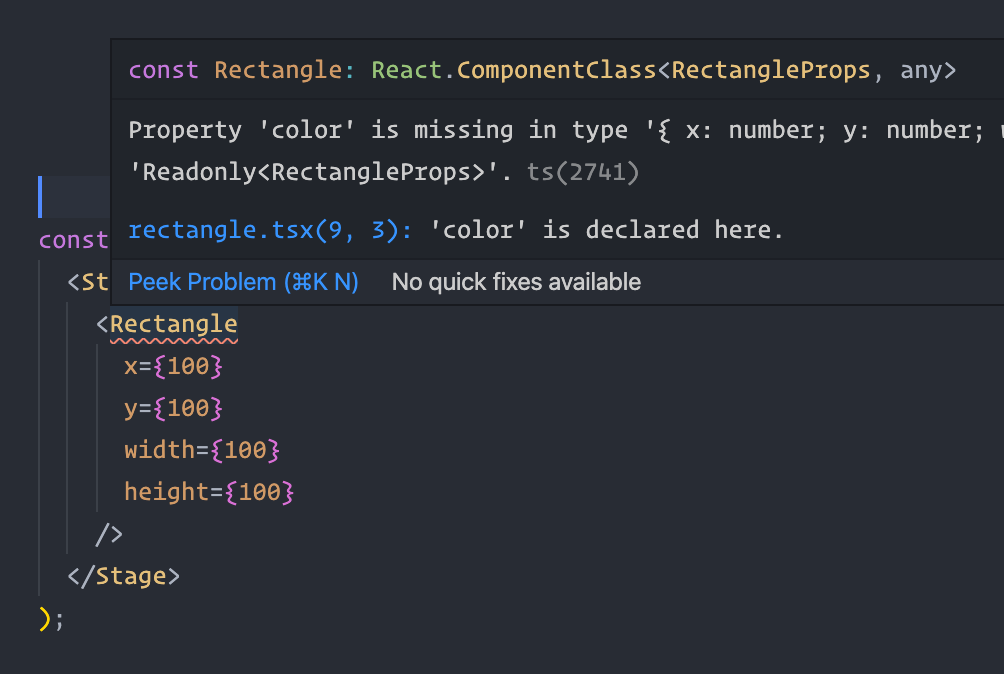
PixiRef
Get the PixiJS instance type for a ReactPixi component:
Example:
import { Container, PixiRef } from '@pixi/react';
type IContainer = PixiRef<typeof Container>; // Pixi Container
const App = () => {
const containerRef = React.useRef<IContainer>(null);
return <Container ref={containerRef} />;
};
Type component props
Extract a component prop type as follow:
import { useCallback, ComponentProps } from 'react';
import { Graphics } from '@pixi/react';
type Draw = ComponentProps<typeof Graphics>['draw'];
const App = () => {
const draw = useCallback<Draw>((g) => {
g; // PIXI.Graphics
g.clear();
g.beginFill(props.color);
g.drawRect(0, 0, 100, 100);
g.endFill();
});
return <Graphics draw={draw} />;
};