About
Pixi React
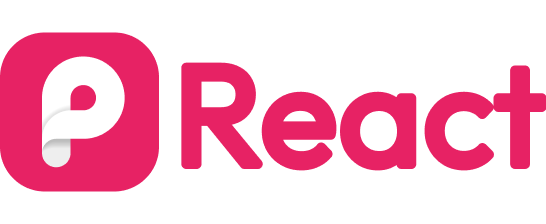
Simply the best way to write PixiJS applications in React
Write PixiJS applications using React declarative style 👌
Write PixiJS applications using React declarative style 👌
 Â
 Â
Features​
- React 17 and 18 support
- PixiJS v6 and v7 support
react-spring
compatible animated components
Quick start​
If you want to start a new React project from scratch, we recommend Vite. To add to an existing React application, just install the dependencies.
1. Create a new React project with Vite:​
# for typescript use "--template react-ts"
npx create-vite@latest --template react my-app
cd my-app
npm install
2. Install Pixi-React dependencies:​
npm install pixi.js@7 @pixi/react@7
3. Replace App.jsx with the following:​
import './App.css';
import { useMemo } from 'react';
import { BlurFilter, TextStyle } from 'pixi.js';
import { Stage, Container, Sprite, Text } from '@pixi/react';
const App = () => {
const blurFilter = useMemo(() => new BlurFilter(2), []);
const bunnyUrl = 'https://pixijs.io/pixi-react/img/bunny.png';
return (
<Stage width={800} height={600} options={{ background: 0x1099bb }}>
<Sprite image={bunnyUrl} x={300} y={150} />
<Sprite image={bunnyUrl} x={500} y={150} />
<Sprite image={bunnyUrl} x={400} y={200} />
<Container x={200} y={200}>
<Text
text="Hello World"
anchor={0.5}
x={220}
y={150}
filters={[blurFilter]}
style={
new TextStyle({
align: 'center',
fill: '0xffffff',
fontSize: 50,
letterSpacing: 20,
dropShadow: true,
dropShadowColor: '#E72264',
dropShadowDistance: 6,
})
}
/>
</Container>
</Stage>
);
};
export default App;
4. Run the app:​
npm run dev
The final result should look like this:
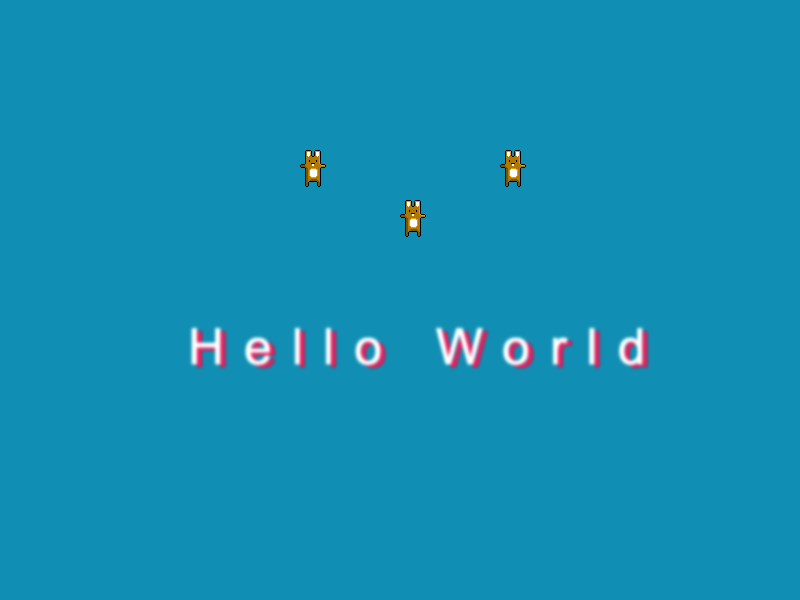
See the Codepen examples
Example​
Result
Loading...
Live Editor
Components​
Pass PixiJS properties directly as component props, example:
import { Sprite } from '@pixi/react';
import { BLEND_MODES, BlurFilter } from 'pixi.js';
import { useMemo } from 'react';
const MyComponent = () => (
const blurFilter = useMemo(() => new BlurFilter(4), []);
<Sprite
texture={myTexture}
anchor={0.5}
position={[100, 200]}
blendMode={BLEND_MODES.ADD}
roundPixels={true}
filters={[blurFilter]}
/>;
);
FAQ​
Interaction does not work
If you are using PixiJS without the pixi.js
package and are instead using scoped packages like @pixi/app
, @pixi/sprite
etc, you will need to import the @pixi/events
package to enable interaction.
import '@pixi/events';
Join us​
You're missing an amazing feature? Or just want to get in touch with fellow developers and have a chat? Feel free to join our Discord channel.