Spine
AssetPack provides several plugins for transforming spine files that utilise atlas/png
format
Spine Atlas Compress
The spineAtlasCompress
plugin uses the Sharp library to compress images into different formats, such as JPEG, PNG, WebP, and AVIF. This helps reduce file sizes while maintaining image quality, ensuring faster load times and better performance.
This plugin also supports compressing images using the ASTC, ETC, ETC2, BCn (DXTn) and Basis supercompressed (ETC1S, UASTC) texture compression standard.
This plugin should be used in conjunction with the compress plugin to ensure that the atlas file is compressed as well as the images.
Example
Original
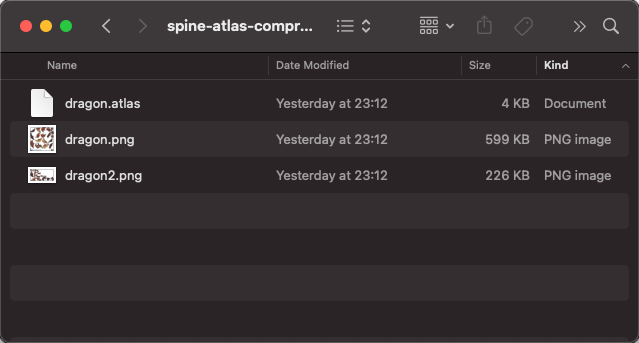
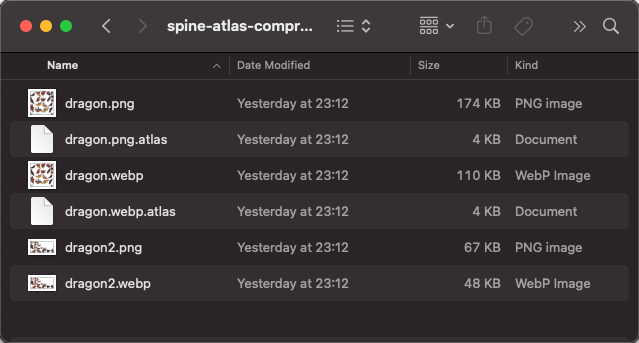
import { compress } from "@assetpack/core/image";
import { spineAtlasCompress } from "@assetpack/core/spine";
// these options are the default values, all options shown here are optional
const options = {
jpg: {},
png: { quality: 90 },
webp: { quality: 80, alphaQuality: 80, },
avif: false,
bc7: false,
astc: false,
basis: false,
etc: false
};
export default {
...
pipes: [
...
compress(options),
spineAtlasCompress(options),
]
};
API
See Compression API for more information.
You will want to make sure you are passing the same options to the compress
plugin as you are to the spineAtlasCompress
plugin.
Tags
Tag | Folder/File | Description |
---|---|---|
nc | both | If present the atlas will not be compressed. |
Spine Atlas Mipmap
The spineAtlasMipmap
plugin generates mipmaps for images, creating multiple resolutions of the same image. This is particularly useful for optimizing graphics in web games, where different resolutions are needed for various screen sizes and device capabilities.
This plugin should be used in conjunction with the mipmap plugin to ensure that the atlas file is mipped as well as the images.
API
See Mipmaps API for more information.
You will want to make sure you are passing the same options to the mipmap
plugin as you are to the spineAtlasMipmap
plugin.
Example
Original
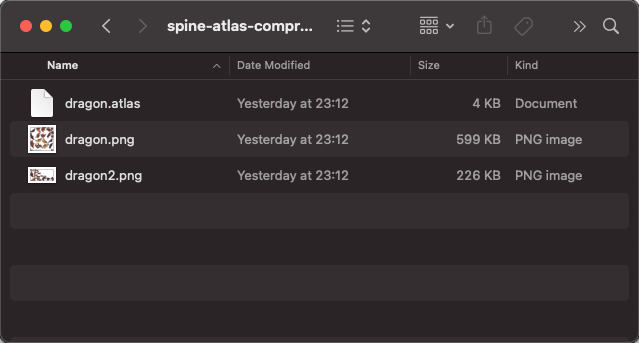
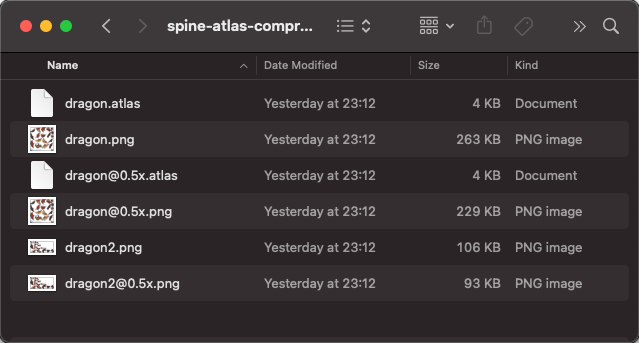
import { mipmap } from "@assetpack/core/image";
import { spineAtlasMipmap } from "@assetpack/core/spine";
// these options are the default values, all options shown here are optional
const options = {
template: "@%%x",
resolutions: { default: 1, low: 0.5 },
fixedResolution: "default",
};
export default {
...
pipes: [
...
mipmap(options),
spineAtlasMipmap(options),
]
};
Tags
Tag | Folder/File | Description |
---|---|---|
fix | both | If present the fixedResolution will be used. No other resolutions will be generated. Note that the image will be resized to the resolution specified in fixedResolution . If you don't want the image to be resized please use the nomip tag. |
nomip | both | If present mipmaps will not be generated. |
Spine Atlas Manifest Mod
The spineAtlasManifestMod
plugin augments the manifest file generated by the manifest plugin to include the spine atlas files.
This plugin should be used in conjunction with the manifest plugin to ensure that the spine atlas files are included in the manifest.
API
N/A for this plugin.
Example
import { pixiManifest } from "@assetpack/core/manifest";
import { spineAtlasManifestMod } from "@assetpack/core/spine";
export default {
...
pipes: [
...
pixiManifest(),
spineAtlasManifestMod(),
]
};
Tags
N/A for this plugin.